Working with Large Datasets in MATLAB
Learn expert techniques for handling large datasets in MATLAB. Optimize memory, enhance processing speed, and use the best tools for efficient data analysis.

Handling large datasets efficiently is a crucial aspect of data analysis, scientific computing, and engineering applications. MATLAB offers powerful tools to work with extensive data while maintaining performance and accuracy. This blog will explore best practices, expert techniques, and top strategies for managing large datasets in MATLAB, ensuring seamless processing and computation.
Why Work with Large Datasets in MATLAB?
MATLAB is a preferred choice for handling large datasets due to its:
-
Optimized matrix operations
-
High-performance computing capabilities
-
Extensive built-in functions for data handling
-
Ability to integrate with other programming environments
Challenges in Handling Large Datasets
Working with large datasets presents several challenges, including:
-
Memory limitations
-
Computational efficiency
-
Data storage and retrieval
-
Processing time optimization
Understanding these challenges helps professionals develop strategies to optimize MATLAB’s performance.
Optimizing MATLAB for Large Dataset Processing
1. Memory Management Techniques
Managing memory effectively is crucial when working with extensive datasets. Consider the following techniques:
Use Efficient Data Types
Choosing appropriate data types reduces memory usage. MATLAB experts recommend using:
-
Single instead of double for floating-point numbers when high precision isn't required.
-
Integer types for categorical or index-based data.
-
Sparse matrices when dealing with large datasets with many zeros.
Clearing Unused Variables
Using clear
for unnecessary variables helps free up memory:
clear variable_name;
Additionally, using pack
compacts workspace memory.
2. Data Import and Storage Best Practices
Efficient data import and storage improve MATLAB’s performance when handling large datasets.
Use MAT-Files for Storage
MAT-Files optimize data storage while maintaining easy access:
save('large_data.mat', 'variable_name', '-v7.3');
The -v7.3
option enables efficient data compression and partial loading.
Read and Write Large Files Efficiently
For reading CSV or text files, readtable
and datastore
functions are recommended:
tbl = readtable('large_data.csv', 'PreserveVariableNames', true);
Using datastore
allows handling data in chunks:
ds = datastore('large_data.csv');
3. Optimizing Data Processing
Efficient data processing ensures smooth handling of large datasets.
Vectorization for Speed
Replacing loops with vectorized operations enhances speed:
A = rand(10000,1);
B = A .* 2; % Vectorized operation instead of loop
Parallel Computing
Parallel computing speeds up processing by distributing tasks across multiple cores:
parpool;
parfor i = 1:1000
result(i) = computeFunction(data(i));
end
Seeking expert help for your matrix algebra assignment writing ? We’re here to assist with every step!
Chunk Processing
Processing data in smaller chunks prevents memory overload:
for i = 1:100:10000
batch = data(i:i+99);
processBatch(batch);
end
4. Data Visualization for Large Datasets
Visualizing large datasets can be computationally expensive. MATLAB offers methods to optimize visualization:
Downsampling Data
Using downsample
reduces plot complexity:
downsampled_data = downsample(large_data, 10);
plot(downsampled_data);
Using Efficient Plotting Functions
For large datasets, scatter
and line
plots work better than plot3
:
scatter(x, y, '.');
MATLAB Tools for Handling Large Datasets
1. Big Data Processing with Tall Arrays
MATLAB’s tall
arrays handle out-of-memory data efficiently:
tallArray = tall(ds);
summary(tallArray);
2. Using MapReduce
mapreduce
is beneficial for processing extensive datasets in a distributed manner:
outds = mapreduce(ds, @mapFun, @reduceFun);
3. Integration with Databases
For very large datasets, MATLAB allows direct connection to SQL databases:
conn = database('myDatabase','username','password');
data = fetch(conn, 'SELECT * FROM largeTable');
Best Practices for Working with Large Datasets
Following best practices ensures smooth operations:
-
Use memory-efficient data structures
-
Utilize parallel computing
-
Read and process data in chunks
-
Optimize code for performance
-
Choose efficient visualization techniques
Conclusion
Handling large datasets in MATLAB requires optimized memory management, efficient processing techniques, and the right tools. By leveraging best practices and expert strategies, professionals can maximize MATLAB’s capabilities while ensuring smooth and efficient data processing. Whether using parallel computing, tall
arrays, or database integration, MATLAB remains one of the best environments for handling extensive datasets with ease.
What's Your Reaction?
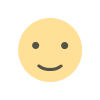

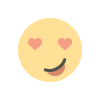

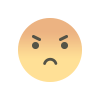
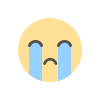
